If you are building a huge infrastructure with Terraform, considering multiple Terraform Workspace is crucial. A Terraform workspace provides you with a separate virtual space to store your persistent data.
Throughout this tutorial, you’ll learn how Terraform workspace lets team members or developers work on a code without impacting existing resources.
Sounds interesting? Read on!
Prerequisites
If you’d like to follow along in this tutorial, ensure you have the following in place:
- An Amazon Web Service account (AWS) – You can create a free-tier account if you don’t have one.
- Terraform v0.14.9 – This tutorial’s demonstrations are on Ubuntu 18.04.5 LTS, but other operating systems with Terraform will work.
- A code editor – Even though you can use any text editor to work with Terraform configuration files, you should have one that understands the HCL Terraform language. Try out Visual Studio (VS) Code.
Creating Multiple Terraform Workspaces
In today’s automation era, engineers such as Terraform developers need multiple workspaces to test the same scripts and code before building rock-solid resources.
Currently, the following backends support multiple workspaces: AzureRM, Consul, COS, GCS, Kubernetes, Local, Manta, Postgres, Remote, and S3.
To better understand how multiple Terraform workspaces work, create two different workspaces:
1. Open your terminal and run the commands below to create a folder in your home directory, and change the working directory to that folder.
In this tutorial, the folder is called terraform-workspace-demo, stored in your home directory. You can name the folder differently as you prefer. This folder stores your Terraform configuration files.
mkdir ~/terraform-workspace-demo
cd ~/terraform-workspace-demo
2. Next, open your favorite code editor, and copy/paste the command below.
The terraform workspace new
command creates a new workspace (workspace1
) and switches your current workspace to the newly created workspace.
For this example, the workspace is named workspace1
, but you can preferably name it anything.
# Creating the new workspace workspace1 and switching to it.
terraform workspace new workspace1

Perhaps you want to switch from one workspace to another. If so, run the command below. Replace
workspace-name
with the actual workspace name.terraform workspace select workspace-name
3. Similarly, run the below command to create another workspace. This time you’ll create another workspace named workspace2.
# Creating the new workspace workspace2 and switching to it.
terraform workspace new workspace2
As you can see below, you created and switched to workspace2 successfully.

If you prefer to delete a workspace you don’t need anymore, run this
terraform workspace delete workspace-name
command.
Building Terraform Configurations to Launch EC2 Instances
You now have two different Terraform workspaces to work with the same Terraform configurations, but you still need to create an instance. You’ll create the terraform configuration file to create an AWS EC2 instance in two different workspaces.
1. Open your favorite code editor, and copy/paste the configuration below. Save the configuration as main.tf inside the ~/terraform-workspace-demo directory.
Terraform uses several different types of configuration files. Each file is written in either plain text or JSON format with a specific naming convention of either .tf or .tfjson format.
The main.tf file allows you to create AWS EC2 instances with the AMI and [instance type] declared as variables. These variables have their values defined in another file named terraform.tfvars that you will create later in this section.
You can find Linux AMIs via the Amazon EC2 console.
# Declaring the resource block aws_instance
resource "aws_instance" "my-machine" {
# Declaring the argument ami
ami = var.ami
# Declaring the argument instance_type
instance_type = var.instance_type
# Tagging the instances
tags = {
Name = "ec2-instance-${terraform.workspace}"
}
}
2. Now, create another file named vars.tf inside the ~/terraform-workspace-demo directory, and copy/paste the content below to the vars.tf file. The vars.tf file is a Terraform variables file, which contains all the variables that the configuration file references.
# Creating a Variable for ami
variable "ami" {
type = string
}
# Creating a Variable for instance_type
variable "instance_type" {
type = string
}
3. Create one more file called provider.tf inside ~/terraform-workspace-demo directory and put in the code below to the provider.tf file. The provider.tf file is where you define the AWS provider so that Terraform can connect to the correct cloud services.
Including all configuration values in a single configuration file is possible. But to keep things clear for developers and admins, breaking the logic and variables into separate files is much more preferable.
The code below defines the AWS provider named aws
and creates resources in the us-east-2
region.
You can launch aws resources in any regions
provider "aws" {
region = "us-east-2"
}
4. Create one last file inside the ~/terraform-workspace-demo directory. Name the file as terraform.tfvars, and paste in the code below. The terraform.tfvars contains the values Terraform uses to replace the variable references inside a configuration file.
ami = "ami-0742a572c2ce45ebf"
instance_type = "t2.micro"
5. Finally, run the tree
command below to verify all of the required files are contained in the terraform-workspace-demo folder.
tree terraform-workspace-demo
Below, you will notice that another directory (terraform.tfstate.d
) contains two folders (workspace1 and workspace2). Terraform automatically creates these folders as you create the Terraform workspace.
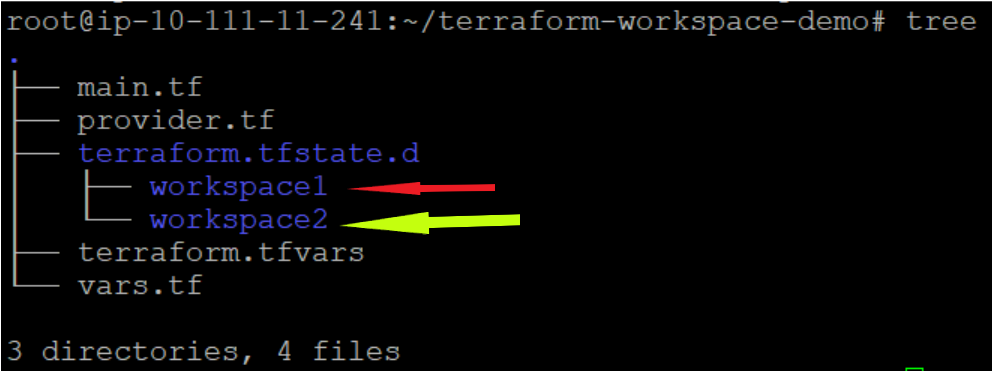
Creating Two AWS EC2 Instances in Different Workspaces
You now have the Terraform workspaces and Terraform configuration files ready, so the next step is to launch AWS EC2 instances. You’ll create and launch two AWS instances within two different workspaces.
1. Run the command below to verify the Terraform workspace before you create resources in the AWS account.
# Displays the current workspace
terraform workspace show
Below, you can see the current workspace is workspace2.

2. Next, run each command below to navigate to ~\\terraform-workspace-demo
directory and initialize Terraform. Terraform initializes the plugins and providers which are required to work with resources.
Terraform typically uses a three-stage approach, which is terraform init → terraform plan → terraform apply.
cd ~\terraform-workspace-demo
## Initializing Terraform
terraform init
## Gives you an overview of which resources will be provisioned in your infrastructure
terraform plan
## Tells Terraform to provision the instance
terraform apply
If all goes well after running terraform apply
command, you’ll get the Apply complete! Resources: 1 added, 0 changed, 0 destroyed message shown below.
Note the output instance ID as you’ll need them to verify if they exist in the AWS Management Console later. Below, you can see the command displays the output’s EC2 instance ID.`

3. Now, run the command below to switch workspace from workspace2 to workspace1. Replace workspace-name
with the workspace’s name you want to switch with the current workspace.
terraform workspace select workspace-name

Finally, rerun the commands below as you previously did (step two). This time, Terraform creates the instance in a different workspace (workspace1).

Verifying the Resources Exist in AWS Console
By now, you should have created all the EC2 instances launched with Terraform. But you can verify if all the EC2 instances exist by manually checking in the AWS Management Console.
1. Open your favorite web browser and log on to the AWS Management Console.
2. Now click on the search bar at the top, search for ‘EC2’, and click on the EC2 menu item below.
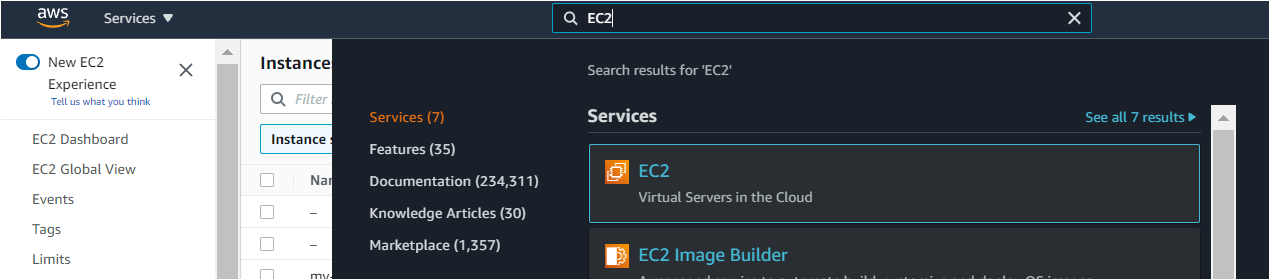
Finally, on the EC2 page, verify that all IDs are the same ones that Terraform returned earlier under the “Creating two AWS EC2 instances in different Workspaces” section.
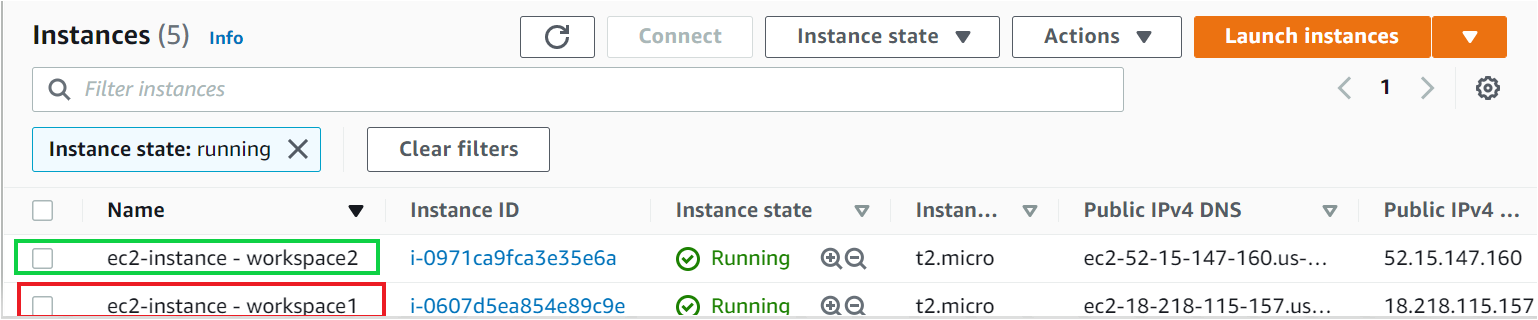
Conclusion
In this tutorial, you’ve learned how to use Terraform workspace to launch unique resources simultaneously using the same Terraform configuration. Now you can work on different workspaces without messing things up!
With this knowledge, take what you have learned and use Terraform to manage your entire infrastructure!